CS 347: Lab 21 – GitHubber
Dear students:
Welcome to lab. Now’s your chance to apply the ideas you read about. Find a partner and complete as much of the task below as you can. At the end of our time together, paste your files into Crowdsource in order to receive credit.
Task 1
Your task is to write a React/Redux app that shows GitHub repositories matching a query. The matches will be provided by GitHub’s REST API. The end product will look something like this:
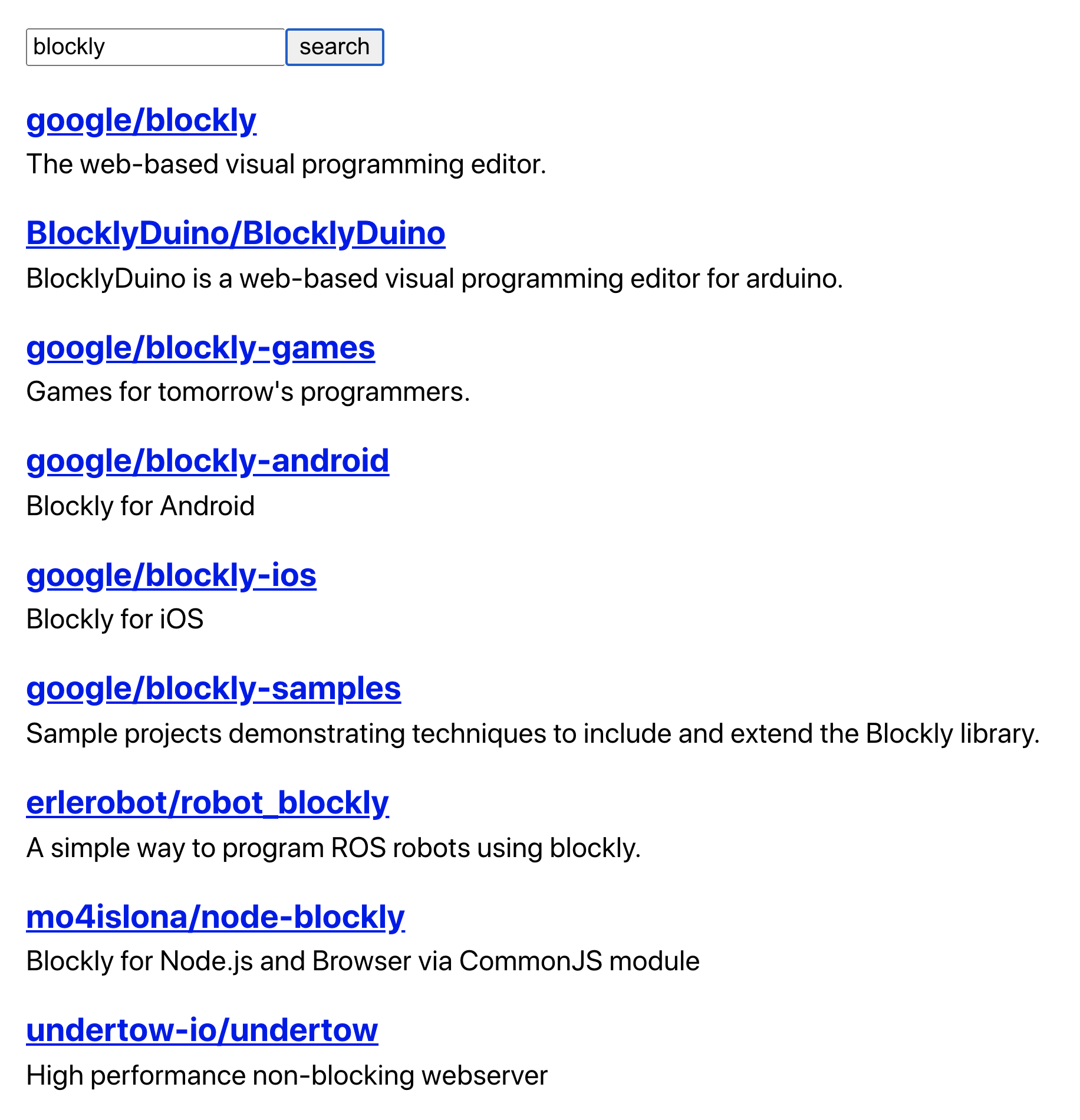
In the terminal, create a new React app and move into its directory:
npx create-react-app githubber cd githubber npm install redux react-redux redux-thunk
The next three sections can be done in parallel. Split them up between team members.
Store
Create store.js
as was done in the reading. Define a reducer that accepts the current state and an action and yields the new state. For the moment, the reducer should not do anything interesting. Whatever the action, return the unmodified state.
For initial testing, prime your state with a structure like this:
const initialState = {
matches: [
{
full_name: 'twodee/fiction',
description: 'This repository does not exist.',
html_url: 'https://github.com/twodee/fiction',
},
],
};
Define a store using the reducer, this initial state, and the thunk
middleware.
Provider
In index.js
, add a Provider
component and pass your store as a prop.
Repository
Define a Repository
component in Repository.js
. It receives a prop named repository
, which is an object shaped like the example from the initial state shown above, having keys full_name
, description
, and html_url
. Display the repository’s full name in an anchor tag and the repository’s description.
App
Your next task is to reach into the global store and turn its matches
array into viewable components. Complete the following steps in App.js
.
- Use the
useSelector
hook to grab the matches from the store. - Map each match to a
Repository
component. You should see the initial state showingtwodee/fiction
.
Next add a search box so the user can query for real repositories. Follow these steps:
- Add a text input for the query.
- Add local state to hold the query text.
- Add a button to fire off the query.
- Add a callback for the button that dispatches a
startSearching
action (defined elsewhere).
Actions
Now that you’ve got the initial state showing, it’s time to add some actions to pull down new state from GitHub’s REST API. Complete the following steps.
- Create
actions.js
. It will have a structure similar to the ones from the readings. - Add an object named
Action
that acts as an enum. Give it an entry forLoadMatches
. - Add an action creator named
loadMatches
that accepts an array of matches as a parameter. Have it return an object whosetype
isAction.LoadMatches
and whosepayload
is the array of matches. - Add a thunk creator named
startSearching
that accepts a query string. It returns a lambda that accepts the dispatch function and fetches a list of repositories that match the query. Use the URLhttps://api.github.com/search/repositories?q=QUERY
, replacingQUERY
with the actual query string. - Interpret the fetched results as JSON. The JSON has the structure specified under Default Response in this endpoint’s documentation. You can also see what is fetched by pasting the URL in your browser’s location bar.
- Once the JSON is parsed, dispatch a
loadMatches
action, passing in the matching repositories. - In your reducer, add a case for
Action.LoadMatches
. Return a new state whosematches
property is the action’s payload.
When the user types in a query and hits the button, the fetch will update the store and automatically re-render the app and display the matching repositories.
TODO
The next step of your learning is to complete the following tasks:
- Read the chapter Multi-page Applications in All Over the Web. Be sure to follow along with the code samples. Recreate each exercise yourself.
- Research some aspect of web development on your own. Find articles and videos beyond what’s assigned. Summarize what you learn in a couple paragraphs of your own words and a snippet of source code in your next blog entry before Friday morning. Clearly put the date of the blog entry on your index page. If any of the requirements is not met, you will not receive full credit.
- Keep working project 3.
See you next time.
